【用 JS 寫一個 Discord Bot!】02 音樂機器人 (舊版)
[公告]
HI 大家好,後來我實際使用這個版本的機器人後,發現播放穩定度不太行,所以我寫了一個新的版本:
【用 JS 寫一個 Discord Bot!】03 Discord.js v14 音樂機器人 (新版)
另外,這個版本已經不推薦使用,就當成紀念封存了。
原文
點我展開
今天我們來寫一個具有以下功能的音樂機器人:
- 播放 YouTube 歌曲
- 暫停/恢復播放
- 跳過歌曲
- 歌曲隊列
如果還不知道怎麼建立機器人,可以參考我之前寫的這篇文章:【用 JS 寫一個 Discord Bot!】01 建立機器人。
實作
必備:
- Node.js 版本 v14.0.0 以上
- 編輯器 (推薦 Visual Studio Code)
安裝套件
Discord 機器人的核心套件
1
| $ npm install discord.js@12.5.3
|
執行音樂的轉檔、串流功能
1
| $ npm install ffmpeg-static
|
Opus 編碼器
1
| $ npm install @discordjs/opus
|
執行下載 YouTube 影片的功能
開始編寫
建立 config.json
在開始之前,我們先在專案中建立 config.json,放一些常用配置。
prefix
設定指令的前綴字。
編寫 discord.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276
| const { Client } = require('discord.js'); const ytdl = require('ytdl-core'); const { token } = require('./token.json'); const { prefix } = require('./config.json'); const client = new Client();
class Music {
constructor() {
this.isPlaying = {};
this.queue = {};
this.connection = {};
this.dispatcher = {}; }
async join(msg) {
if (msg.member.voice.channel !== null) { this.connection[msg.guild.id] = await msg.member.voice.channel.join(); } else { msg.channel.send('請先進入語音頻道'); }
}
async play(msg) {
const guildID = msg.guild.id;
if (!this.connection[guildID]) { msg.channel.send('請先將機器人 `!!join` 加入頻道'); return; }
if (this.connection[guildID].status === 4) { msg.channel.send('請先將機器人 `!!join` 重新加入頻道'); return; }
const musicURL = msg.content.replace(`${prefix}play`, '').trim();
try {
const res = await ytdl.getInfo(musicURL); const info = res.videoDetails;
if (!this.queue[guildID]) { this.queue[guildID] = []; }
this.queue[guildID].push({ name: info.title, url: musicURL });
if (this.isPlaying[guildID]) { msg.channel.send(`歌曲加入隊列:${info.title}`); } else { this.isPlaying[guildID] = true; this.playMusic(msg, guildID, this.queue[guildID][0]); }
} catch(e) { console.log(e); }
}
playMusic(msg, guildID, musicInfo) {
msg.channel.send(`播放音樂:${musicInfo.name}`);
this.dispatcher[guildID] = this.connection[guildID].play(ytdl(musicInfo.url, { filter: 'audioonly' }));
this.dispatcher[guildID].setVolume(0.5);
this.queue[guildID].shift();
this.dispatcher[guildID].on('finish', () => {
if (this.queue[guildID].length > 0) { this.playMusic(msg, guildID, this.queue[guildID][0]); } else { this.isPlaying[guildID] = false; msg.channel.send('目前沒有音樂了,請加入音樂 :D'); }
});
}
resume(msg) {
if (this.dispatcher[msg.guild.id]) { msg.channel.send('恢復播放');
this.dispatcher[msg.guild.id].resume(); }
}
pause(msg) {
if (this.dispatcher[msg.guild.id]) { msg.channel.send('暫停播放');
this.dispatcher[msg.guild.id].pause(); }
}
skip(msg) {
if (this.dispatcher[msg.guild.id]) { msg.channel.send('跳過目前歌曲');
this.dispatcher[msg.guild.id].end(); }
}
nowQueue(msg) {
if (this.queue[msg.guild.id] && this.queue[msg.guild.id].length > 0) { const queueString = this.queue[msg.guild.id].map((item, index) => `[${index+1}] ${item.name}`).join(); msg.channel.send(queueString); } else { msg.channel.send('目前隊列中沒有歌曲'); }
}
leave(msg) {
if (this.connection[msg.guild.id] && this.connection[msg.guild.id].status === 0) {
if (this.queue.hasOwnProperty(msg.guild.id)) {
delete this.queue[msg.guild.id];
this.isPlaying[msg.guild.id] = false; }
this.connection[msg.guild.id].disconnect(); } else { msg.channel.send('機器人未加入任何頻道'); }
} }
const music = new Music();
client.on('message', async (msg) => {
if (!msg.guild) return;
if (msg.content === `${prefix}join`) {
music.join(msg); }
if (msg.content.indexOf(`${prefix}play`) > -1) {
if (msg.member.voice.channel) {
await music.play(msg); } else {
msg.reply('你必須先加入語音頻道'); } }
if (msg.content === `${prefix}resume`) {
music.resume(msg); }
if (msg.content === `${prefix}pause`) {
music.pause(msg); }
if (msg.content === `${prefix}skip`) {
music.skip(msg); }
if (msg.content === `${prefix}queue`) {
music.nowQueue(msg); }
if (msg.content === `${prefix}leave`) {
music.leave(msg); } });
client.on('ready', () => { console.log(`Logged in as ${client.user.tag}!`); });
client.login(token);
|
寫完後就可以運行看看。
操作流程是先 !!join
讓機器人加入語音頻道→ !!play 音樂網址
播放音樂或加入隊列(如果音樂正在播放)。
功能如下:
- 【機器人加入語音】
!!join
- 【播放音樂(加入隊列)】
!!play 音樂網址
- 【暫停播放】
!!pause
- 【恢復播放】
!!resume
- 【跳過這首歌曲】
!!skip
- 【查看歌曲隊列】
!!queue
- 【讓機器人離開語音頻道(會清空歌曲隊列)】
!!leave
可以自行玩玩看。
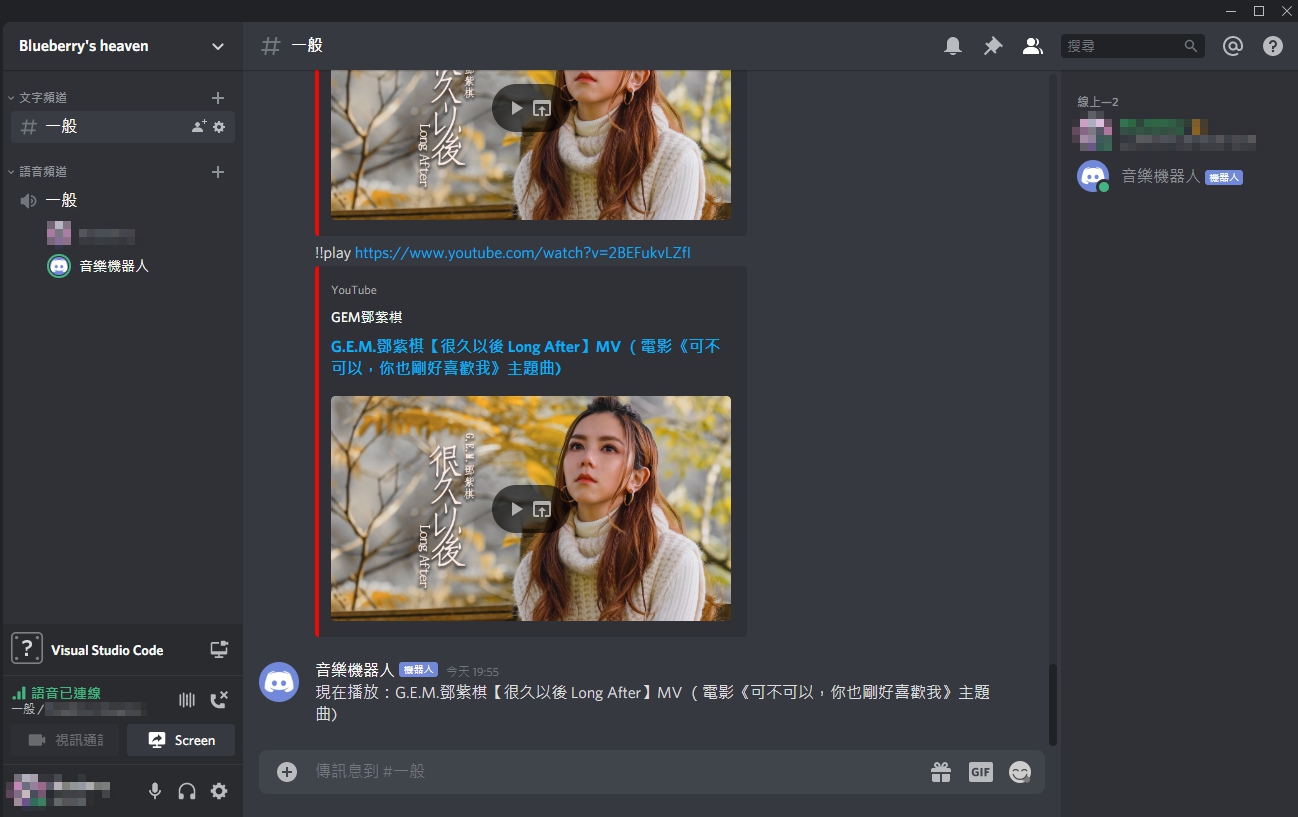
本來想把程式碼切開在文中講解,但是發現這樣寫起來會篇幅太長而且雜亂,所以就乾脆把註解寫在 code 裡面。
本次音樂機器人的 Github Repository,可以自行 clone 下來研究或修改。
FAQ
這邊蒐集了可能會遇到的問題。
點我展開
- Q:執行程式後出現
Error: Cannot find module 'xxx'
怎麼辦?
- A:通常那個
xxx
是缺少安裝的套件。跑一下安裝套件的指令 npm i 套件名稱
應該就正常了。
- Q:下 play 指令後機器人跳出
MinigetError: Status code: 404
的錯誤訊息怎麼辦?
- A:請更新 node-ytdl-core 至最新版本。
npm i node-ytdl-core@latest
目前可正常運行的版本(參考用)
- node.js: v14.16
- @discordjs/opus: “0.5.0”
- discord.js: “12.5.3”
- ffmpeg-static: “4.2.8”
- ytdl-core: “4.8.3”
文章更新記錄
點我展開
2022/05/07 更新
棄用。發布新版 → https://b-l-u-e-b-e-r-r-y.github.io/post/DiscordBot02/。
2021/09/22 更新
改為統一使用 v12.5.3 的 discord.js。
2021/06/27 更新
加入一些防呆機制以及更新套件版本。
舊朋友如果有遇到問題,請試著更新 node-ytdl-core 為最新版本(4.8.3):
1
| npm install ytdl-core@latest
|
2021/05/30 更新
發現機器人無法於不同伺服器播放歌曲的問題,是因為原本的 isPlaying
變數忘了寫成物件,變成多個伺服器共用一個變數。
改為:
2021/02/03 更新
發現更新 ytdl 套件後會無法播放音樂的問題,因為 musicURL
字串的空格沒處理乾淨:
1
| const musicURL = msg.content.replace(`${prefix}play`, '');
|
改為:
1
| const musicURL = msg.content.replace(`${prefix}play`, '').trim();
|
2021/01/13 更新
發現之前會有無法播放的問題,已更新 ytdl 套件解決問題。
【用 JS 寫一個 Discord Bot!】系列文章
文章結束囉~
如果我的文章對你有幫助,可以幫我拍個手,感謝支持!